What is IndexOutOfBoundsException (java.lang.IndexOutOfBoundsException)?
IndexOutOfBoundsException
is a runtime exception in the Java programming language. It is thrown when an application attempts to access an array or a string index that is out of bounds. This means that the index is either negative or greater than or equal to the size of the array or string. This exception can occur when using methods such as array[index]
, string.charAt(index)
, or string.substring(beginIndex, endIndex)
. It can also occur when using an iterator to traverse a collection and the iterator goes past the end of the collection.
For example, if you try to access the 10th element of an array that only has 5 elements, an IndexOutOfBoundsException
will be thrown. Similarly, if you try to get the character at index 15 in a string that only has 10 characters, an IndexOutOfBoundsException
will be thrown.
This exception can be thrown when using methods such as array[index]
, string.charAt(index)
, or string.substring(beginIndex, endIndex)
. It can also occur when using an iterator to traverse a collection and the iterator goes past the end of the collection.
It is important to note that, it’s always a good practice to validate the input for the correct range before proceeding with further operation, in order to avoid such kind of exceptions.
Free XML Tools for Android Studio
How to Solve java.IndexOutOfBoundsException?
There are a few ways to solve a java.lang.IndexOutOfBoundsException
:
- Check the array or string index before accessing it: Make sure that the index is greater than or equal to 0 and less than the size of the array or string.
- Use a try-catch block to catch the exception: You can use a try-catch block to catch the exception and handle it gracefully by providing a default value or some other appropriate action.
- Modifying the data structure: The error may occur because of the data structure you are using is not appropriate for the problem or you are using the wrong data structure.
- Using a Collection Framework: If you are working with a collection framework, you can use the
List.get()
method or theIterator.next()
method to avoid the exception, as these methods will throw anIndexOutOfBoundsException
if the index is out of bounds. - Debugging: If the above steps does not solve the problem, use debugging tools like breakpoints, print statements, and a debugger to find the source of the exception and fix it.
It is important to note that, it’s always a good practice to validate the input for the correct range before proceeding with further operation, in order to avoid such kind of exceptions.
Here is an example of a IndexOutOfBoundsException
in Java:
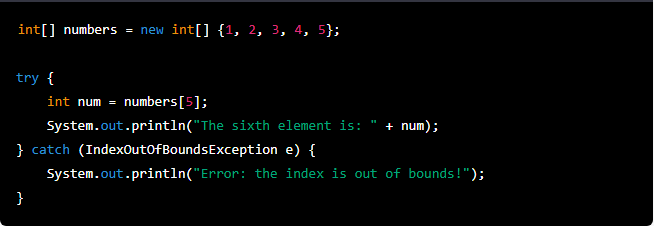
In this example, an array numbers
is created with 5 elements. The program then tries to access the 6th element of the array using the index 5, which is out of bounds (since the array only has 5 elements with indices 0 to 4). This causes the IndexOutOfBoundsException
to be thrown and the catch block is executed, printing out an error message.
Another example would be using a string:

In this example, a string word
is created with 3 characters. The program then tries to access the 4th character of the string using the index 3, which is out of bounds (since the string only has 3 characters with indices 0 to 2). This causes the IndexOutOfBoundsException
to be thrown and the catch block is executed, printing out an error message.
It’s always a good practice to validate the input for the correct range before proceeding with further operation, in order to avoid such kind of exceptions.